- 737
- 2
Сделал слоты, все создается. Но возникает следующий трабл:
Один итем кладется сразу в несколько слотов, но когда вынимаешь любой из них - он остается один. Вот код:
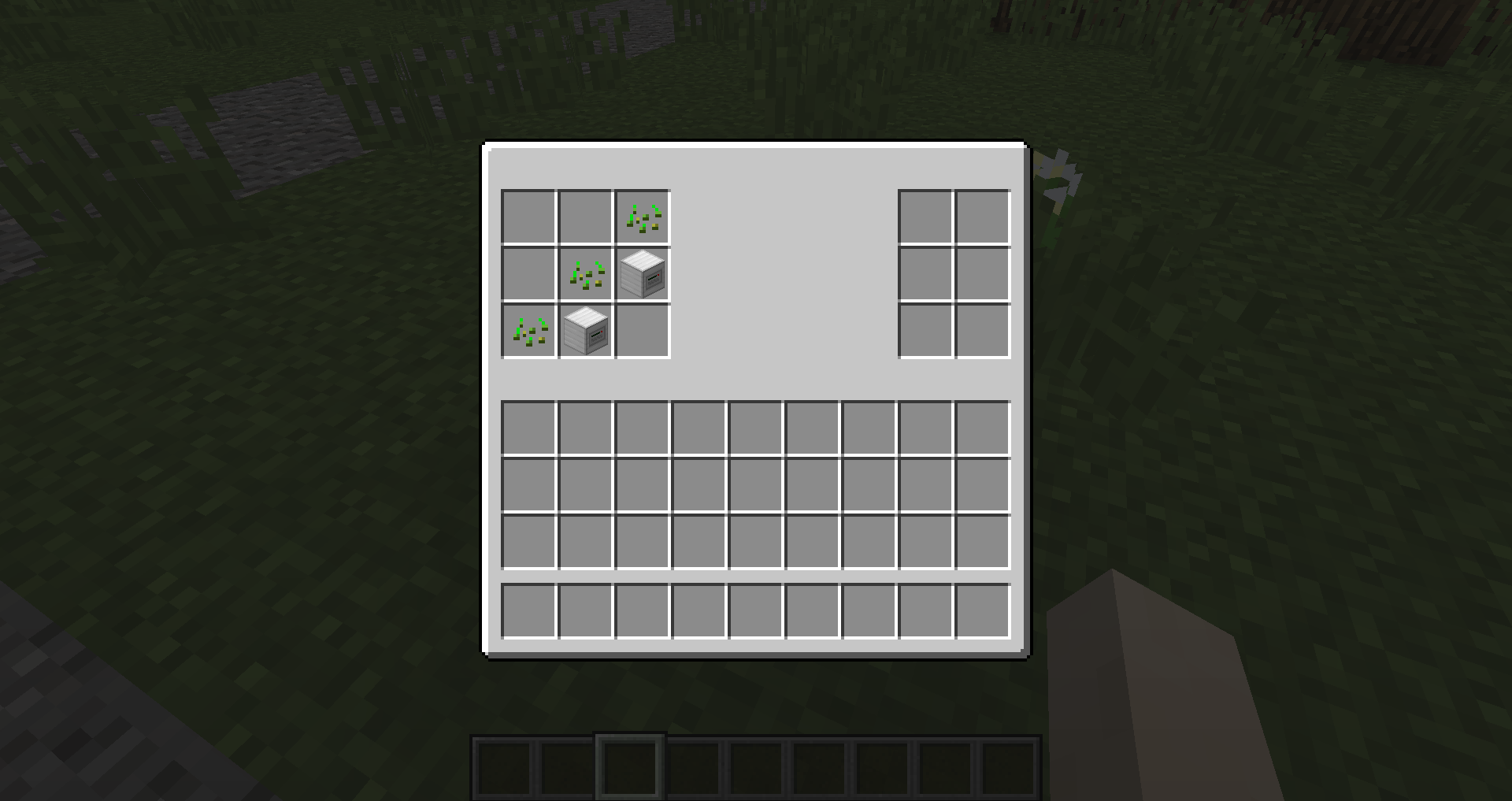
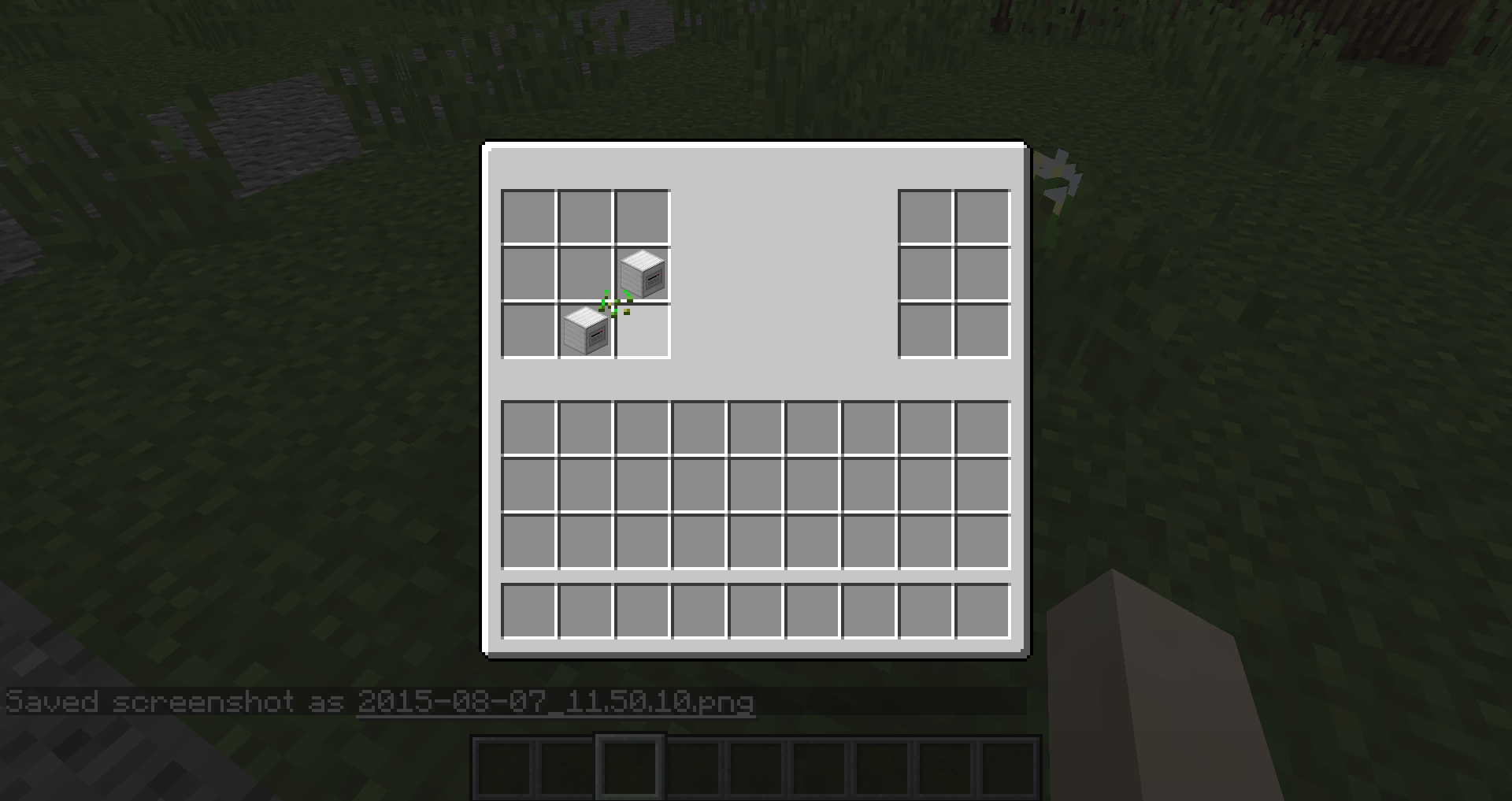
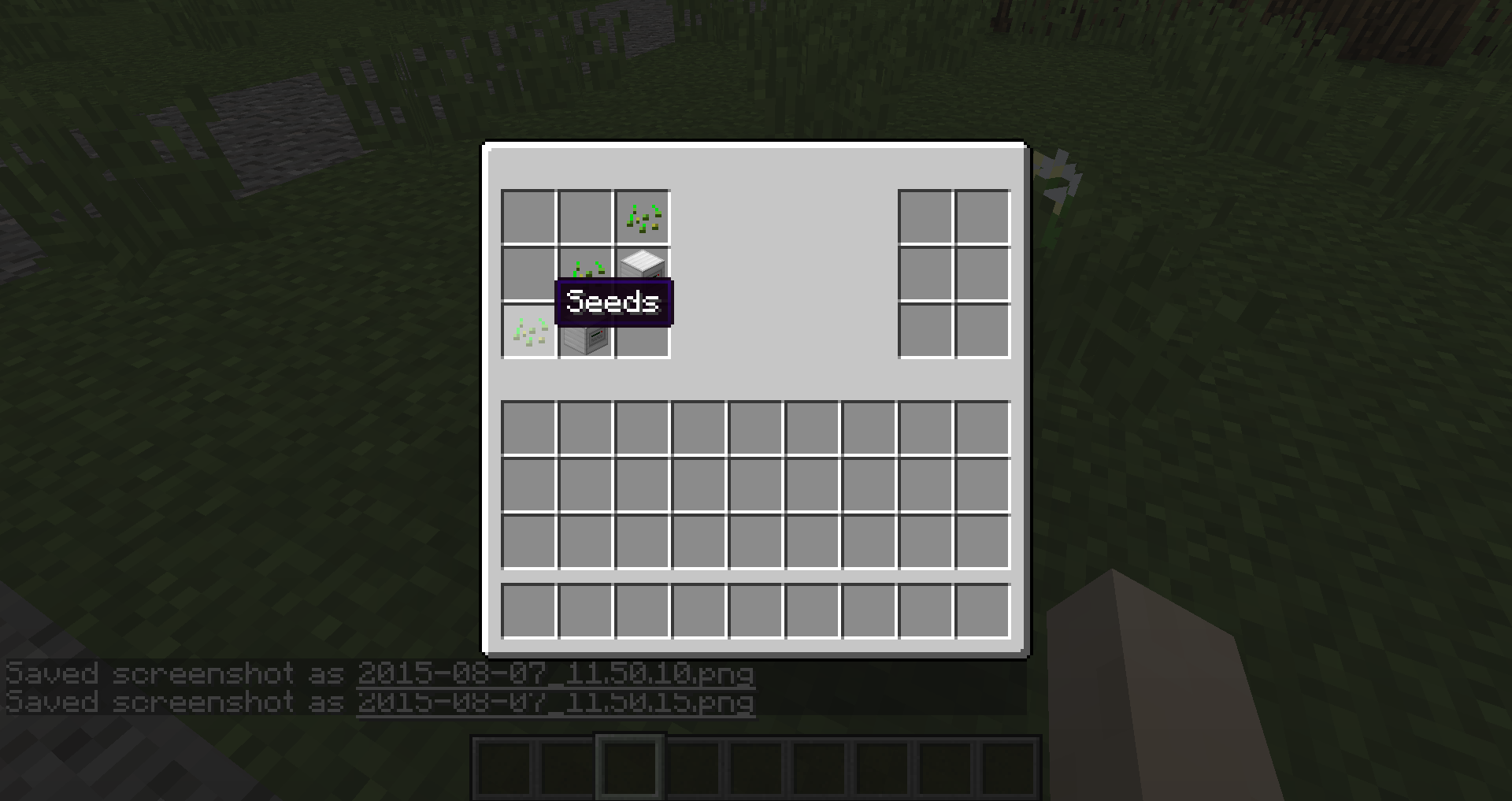
Один итем кладется сразу в несколько слотов, но когда вынимаешь любой из них - он остается один. Вот код:
Код:
public class TETrademat extends TileEntity implements IInventory {
private ItemStack[] tradematContent = new ItemStack[27];
private String customName;
public int numPlayerUsing;
@Override
public int getSizeInventory() {
return 27;
}
@Override
public ItemStack getStackInSlot(int index) {
return this.tradematContent[index];
}
@Override
public ItemStack decrStackSize(int index, int count) {
if (this.tradematContent[index] != null)
{
ItemStack stack;
if (this.tradematContent[index].stackSize <= count)
{
stack = this.tradematContent[index];
this.tradematContent[index] = null;
this.markDirty();
return stack;
}
else
{
stack = this.tradematContent[index].splitStack(count);
if (this.tradematContent[index].stackSize == 0)
{
this.tradematContent[index] = null;
}
this.markDirty();
return stack;
}
}
else
{
return null;
}
}
@Override
public ItemStack getStackInSlotOnClosing(int index) {
if (this.tradematContent[index] != null)
{
ItemStack stack = this.tradematContent[index];
this.tradematContent[index] = null;
return stack;
}
else
{
return null;
}
}
@Override
public void setInventorySlotContents(int index, ItemStack stack) {
this.tradematContent[index] = stack;
if (stack != null && stack.stackSize > this.getInventoryStackLimit())
{
stack.stackSize = this.getInventoryStackLimit();
}
this.markDirty();
}
@Override
public int getInventoryStackLimit() {
return 64;
}
@Override
public boolean isUseableByPlayer(EntityPlayer player) {
return this.worldObj.getTileEntity(this.pos) != this ? false : player.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D;
}
@Override
public void openInventory(EntityPlayer player) {
if (!player.isSpectator())
{
if (this.numPlayerUsing < 0)
{
this.numPlayerUsing = 0;
}
++this.numPlayerUsing;
this.worldObj.addBlockEvent(this.pos, this.getBlockType(), 1, this.numPlayerUsing);
this.worldObj.notifyNeighborsOfStateChange(this.pos, this.getBlockType());
this.worldObj.notifyNeighborsOfStateChange(this.pos.down(), this.getBlockType());
}
}
@Override
public void closeInventory(EntityPlayer player) {
if (!player.isSpectator() && this.getBlockType() instanceof Trademat)
{
--this.numPlayerUsing;
this.worldObj.addBlockEvent(this.pos, this.getBlockType(), 1, this.numPlayerUsing);
this.worldObj.notifyNeighborsOfStateChange(this.pos, this.getBlockType());
this.worldObj.notifyNeighborsOfStateChange(this.pos.down(), this.getBlockType());
}
}
@Override
public boolean isItemValidForSlot(int index, ItemStack stack) {
return true;
}
@Override
public int getField(int id) {
return 0;
}
@Override
public void setField(int id, int value) {
}
@Override
public int getFieldCount() {
return 0;
}
@Override
public void clear() {
for (int i = 0; i < this.tradematContent.length; ++i)
{
this.tradematContent[i] = null;
}
}
@Override
public String getName() {
return this.hasCustomName() ? this.customName : "container.trademat";
}
@Override
public boolean hasCustomName() {
return this.customName != null && this.customName.length() > 0;
}
@Override
public IChatComponent getDisplayName() {
return null;
}
public void readFromNBT(NBTTagCompound compound) {
super.readFromNBT(compound);
NBTTagList list = compound.getTagList("Items", 10);
this.tradematContent = new ItemStack[this.getSizeInventory()];
if (compound.hasKey("CustomName", 8)) {
this.customName = compound.getString("CustomName");
}
for (int i = 0; i < list.tagCount(); i++) {
NBTTagCompound compound1 = list.getCompoundTagAt(i);
int j = compound1.getByte("Slot") & 255;
if (j >= 0 && j < this.tradematContent.length) {
this.tradematContent[j] = ItemStack.loadItemStackFromNBT(compound1);
}
}
}
public void writeToNBT(NBTTagCompound compound)
{
super.writeToNBT(compound);
NBTTagList list = new NBTTagList();
for (int i = 0; i < this.tradematContent.length; ++i)
{
if (this.tradematContent[i] != null)
{
NBTTagCompound compound1 = new NBTTagCompound();
compound1.setByte("Slot", (byte)i);
this.tradematContent[i].writeToNBT(compound1);
list.appendTag(compound1);
}
}
compound.setTag("Items", list);
if (this.hasCustomName())
{
compound.setString("CustomName", this.customName);
}
}
}
Код:
public class ContainerTrademat extends Container {
private TETrademat tile;
private EntityPlayer player;
public ContainerTrademat(EntityPlayer p, TETrademat te)
{
player = p; tile = te;
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
{
addSlotToContainer(new Slot(tile, j + i, 8 + j * 18, 17 + i * 18)); //Trademat contain
}
//addSlotToContainer(new Slot(tile, 0, 8, 17)); //Trademat contain
for (int i = 0; i < 9; i++)
{
addSlotToContainer(new Slot(player.inventory, i, 8 + i * 18, 142));
}
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 9; j++)
{
addSlotToContainer(new Slot(player.inventory, j + i * 9 + 9, 8 + j * 18, 84 + i * 18));
}
}
}
@Override
public ItemStack transferStackInSlot(EntityPlayer player, int index) { return null; }
@Override
public boolean canInteractWith(EntityPlayer player) { return true; }
}