- 2
- 0
Добрый вечер. Я сделал в своём моде аналог ванильного факела, но в игре на его модели не отображается текстура. По обводке видно, что модель регистрируется успешно. На модели предмета (в инвентаре) текстура отображается нормально. Недавно перешёл на 1.12.2, до этого веселился с 1.7.10.
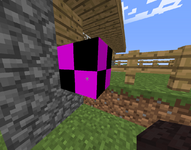

(Если присмотреться, можно увидеть обводку, как у модели стандартного факела)
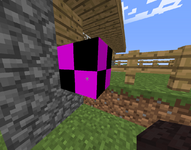

(Если присмотреться, можно увидеть обводку, как у модели стандартного факела)
Класс моего факела (мало чем отличается от ванильного):
package ru.postap;
import java.util.Random;
import javax.annotation.Nullable;
import com.google.common.base.Predicate;
import net.minecraft.block.Block;
import net.minecraft.block.material.Material;
import net.minecraft.block.properties.IProperty;
import net.minecraft.block.properties.PropertyDirection;
import net.minecraft.block.state.BlockFaceShape;
import net.minecraft.block.state.BlockStateContainer;
import net.minecraft.block.state.IBlockState;
import net.minecraft.creativetab.CreativeTabs;
import net.minecraft.entity.EntityLivingBase;
import net.minecraft.util.BlockRenderLayer;
import net.minecraft.util.EnumFacing;
import net.minecraft.util.EnumParticleTypes;
import net.minecraft.util.Mirror;
import net.minecraft.util.Rotation;
import net.minecraft.util.math.AxisAlignedBB;
import net.minecraft.util.math.BlockPos;
import net.minecraft.world.IBlockAccess;
import net.minecraft.world.World;
import net.minecraftforge.fml.relauncher.Side;
import net.minecraftforge.fml.relauncher.SideOnly;
public class NewTorch extends Block {
public static final PropertyDirection FACING = PropertyDirection.create("facing", new Predicate<EnumFacing>()
{
public boolean apply(@Nullable EnumFacing p_apply_1_)
{
return p_apply_1_ != EnumFacing.DOWN;
}
});
protected static final AxisAlignedBB STANDING_AABB = new AxisAlignedBB(0.4000000059604645D, 0.0D, 0.4000000059604645D, 0.6000000238418579D, 0.6000000238418579D, 0.6000000238418579D);
protected static final AxisAlignedBB TORCH_NORTH_AABB = new AxisAlignedBB(0.3499999940395355D, 0.20000000298023224D, 0.699999988079071D, 0.6499999761581421D, 0.800000011920929D, 1.0D);
protected static final AxisAlignedBB TORCH_SOUTH_AABB = new AxisAlignedBB(0.3499999940395355D, 0.20000000298023224D, 0.0D, 0.6499999761581421D, 0.800000011920929D, 0.30000001192092896D);
protected static final AxisAlignedBB TORCH_WEST_AABB = new AxisAlignedBB(0.699999988079071D, 0.20000000298023224D, 0.3499999940395355D, 1.0D, 0.800000011920929D, 0.6499999761581421D);
protected static final AxisAlignedBB TORCH_EAST_AABB = new AxisAlignedBB(0.0D, 0.20000000298023224D, 0.3499999940395355D, 0.30000001192092896D, 0.800000011920929D, 0.6499999761581421D);
protected NewTorch()
{
super(Material.CIRCUITS);
this.setUnlocalizedName("torch_i");
this.setRegistryName("torch_i");
this.setLightLevel(1.0F);
this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.UP));
this.setTickRandomly(true);
this.setCreativeTab(CreativeTabs.DECORATIONS);
}
public AxisAlignedBB getBoundingBox(IBlockState state, IBlockAccess source, BlockPos pos)
{
switch ((EnumFacing)state.getValue(FACING))
{
case EAST:
return TORCH_EAST_AABB;
case WEST:
return TORCH_WEST_AABB;
case SOUTH:
return TORCH_SOUTH_AABB;
case NORTH:
return TORCH_NORTH_AABB;
default:
return STANDING_AABB;
}
}
@Nullable
public AxisAlignedBB getCollisionBoundingBox(IBlockState blockState, IBlockAccess worldIn, BlockPos pos)
{
return NULL_AABB;
}
public boolean isOpaqueCube(IBlockState state)
{
return false;
}
public boolean isFullCube(IBlockState state)
{
return false;
}
private boolean canPlaceOn(World worldIn, BlockPos pos)
{
IBlockState state = worldIn.getBlockState(pos);
return state.getBlock().canPlaceTorchOnTop(state, worldIn, pos);
}
public boolean canPlaceBlockAt(World worldIn, BlockPos pos)
{
for (EnumFacing enumfacing : FACING.getAllowedValues())
{
if (this.canPlaceAt(worldIn, pos, enumfacing))
{
return true;
}
}
return false;
}
private boolean canPlaceAt(World worldIn, BlockPos pos, EnumFacing facing)
{
BlockPos blockpos = pos.offset(facing.getOpposite());
IBlockState iblockstate = worldIn.getBlockState(blockpos);
Block block = iblockstate.getBlock();
BlockFaceShape blockfaceshape = iblockstate.getBlockFaceShape(worldIn, blockpos, facing);
if (facing.equals(EnumFacing.UP) && this.canPlaceOn(worldIn, blockpos))
{
return true;
}
else if (facing != EnumFacing.UP && facing != EnumFacing.DOWN)
{
return !isExceptBlockForAttachWithPiston(block) && blockfaceshape == BlockFaceShape.SOLID;
}
else
{
return false;
}
}
public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer)
{
if (this.canPlaceAt(worldIn, pos, facing))
{
return this.getDefaultState().withProperty(FACING, facing);
}
else
{
for (EnumFacing enumfacing : EnumFacing.Plane.HORIZONTAL)
{
if (this.canPlaceAt(worldIn, pos, enumfacing))
{
return this.getDefaultState().withProperty(FACING, enumfacing);
}
}
return this.getDefaultState();
}
}
public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state)
{
this.checkForDrop(worldIn, pos, state);
}
public void neighborChanged(IBlockState state, World worldIn, BlockPos pos, Block blockIn, BlockPos fromPos)
{
this.onNeighborChangeInternal(worldIn, pos, state);
}
protected boolean onNeighborChangeInternal(World worldIn, BlockPos pos, IBlockState state)
{
if (!this.checkForDrop(worldIn, pos, state))
{
return true;
}
else
{
EnumFacing enumfacing = (EnumFacing)state.getValue(FACING);
EnumFacing.Axis enumfacing$axis = enumfacing.getAxis();
EnumFacing enumfacing1 = enumfacing.getOpposite();
BlockPos blockpos = pos.offset(enumfacing1);
boolean flag = false;
if (enumfacing$axis.isHorizontal() && worldIn.getBlockState(blockpos).getBlockFaceShape(worldIn, blockpos, enumfacing) != BlockFaceShape.SOLID)
{
flag = true;
}
else if (enumfacing$axis.isVertical() && !this.canPlaceOn(worldIn, blockpos))
{
flag = true;
}
if (flag)
{
this.dropBlockAsItem(worldIn, pos, state, 0);
worldIn.setBlockToAir(pos);
return true;
}
else
{
return false;
}
}
}
protected boolean checkForDrop(World worldIn, BlockPos pos, IBlockState state)
{
if (state.getBlock() == this && this.canPlaceAt(worldIn, pos, (EnumFacing)state.getValue(FACING)))
{
return true;
}
else
{
if (worldIn.getBlockState(pos).getBlock() == this)
{
this.dropBlockAsItem(worldIn, pos, state, 0);
worldIn.setBlockToAir(pos);
}
return false;
}
}
@SideOnly(Side.CLIENT)
public void randomDisplayTick(IBlockState stateIn, World worldIn, BlockPos pos, Random rand)
{
EnumFacing enumfacing = (EnumFacing)stateIn.getValue(FACING);
double d0 = (double)pos.getX() + 0.5D;
double d1 = (double)pos.getY() + 0.7D;
double d2 = (double)pos.getZ() + 0.5D;
double d3 = 0.22D;
double d4 = 0.27D;
if (enumfacing.getAxis().isHorizontal())
{
EnumFacing enumfacing1 = enumfacing.getOpposite();
worldIn.spawnParticle(EnumParticleTypes.SMOKE_NORMAL, d0 + 0.27D * (double)enumfacing1.getFrontOffsetX(), d1 + 0.22D, d2 + 0.27D * (double)enumfacing1.getFrontOffsetZ(), 0.0D, 0.0D, 0.0D);
worldIn.spawnParticle(EnumParticleTypes.FLAME, d0 + 0.27D * (double)enumfacing1.getFrontOffsetX(), d1 + 0.22D, d2 + 0.27D * (double)enumfacing1.getFrontOffsetZ(), 0.0D, 0.0D, 0.0D);
}
else
{
worldIn.spawnParticle(EnumParticleTypes.SMOKE_NORMAL, d0, d1, d2, 0.0D, 0.0D, 0.0D);
worldIn.spawnParticle(EnumParticleTypes.FLAME, d0, d1, d2, 0.0D, 0.0D, 0.0D);
}
}
public IBlockState getStateFromMeta(int meta)
{
IBlockState iblockstate = this.getDefaultState();
switch (meta)
{
case 1:
iblockstate = iblockstate.withProperty(FACING, EnumFacing.EAST);
break;
case 2:
iblockstate = iblockstate.withProperty(FACING, EnumFacing.WEST);
break;
case 3:
iblockstate = iblockstate.withProperty(FACING, EnumFacing.SOUTH);
break;
case 4:
iblockstate = iblockstate.withProperty(FACING, EnumFacing.NORTH);
break;
case 5:
default:
iblockstate = iblockstate.withProperty(FACING, EnumFacing.UP);
}
return iblockstate;
}
@SideOnly(Side.CLIENT)
public BlockRenderLayer getBlockLayer()
{
return BlockRenderLayer.CUTOUT;
}
public int getMetaFromState(IBlockState state)
{
int i = 0;
switch ((EnumFacing)state.getValue(FACING))
{
case EAST:
i = i | 1;
break;
case WEST:
i = i | 2;
break;
case SOUTH:
i = i | 3;
break;
case NORTH:
i = i | 4;
break;
case DOWN:
case UP:
default:
i = i | 5;
}
return i;
}
public IBlockState withRotation(IBlockState state, Rotation rot)
{
return state.withProperty(FACING, rot.rotate((EnumFacing)state.getValue(FACING)));
}
public IBlockState withMirror(IBlockState state, Mirror mirrorIn)
{
return state.withRotation(mirrorIn.toRotation((EnumFacing)state.getValue(FACING)));
}
protected BlockStateContainer createBlockState()
{
return new BlockStateContainer(this, new IProperty[] {FACING});
}
public BlockFaceShape getBlockFaceShape(IBlockAccess p_193383_1_, IBlockState p_193383_2_, BlockPos p_193383_3_, EnumFacing p_193383_4_)
{
return BlockFaceShape.UNDEFINED;
}
}
Рендер блока:
@SideOnly(Side.CLIENT)
private static void setRender(Block block) {
Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory"));
}
blockstates/torch_i:
{
"variants": {
"facing=up": { "model": "postap:block/torch_i" },
"facing=east": { "model": "postap:block/torch_i_wall" },
"facing=south": { "model": "postap:block/torch_i_wall", "y": 90 },
"facing=west": { "model": "postap:block/torch_i_wall", "y": 180 },
"facing=north": { "model": "postap:block/torch_i_wall", "y": 270 }
}
}
models/block/torch_i:
{
"ambientocclusion": false,
"textures": {
"particle": "#torch"
},
"elements": [
{ "from": [ 7, 0, 7 ],
"to": [ 9, 10, 9 ],
"shade": false,
"faces": {
"down": { "uv": [ 7, 13, 9, 15 ], "texture": "postap:blocks/torch_i" },
"up": { "uv": [ 7, 6, 9, 8 ], "texture": "postap:blocks/torch_i" }
}
},
{ "from": [ 7, 0, 0 ],
"to": [ 9, 16, 16 ],
"shade": false,
"faces": {
"west": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" },
"east": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" }
}
},
{ "from": [ 0, 0, 7 ],
"to": [ 16, 16, 9 ],
"shade": false,
"faces": {
"north": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" },
"south": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" }
}
}
]
}
models/block/torch_i_wall:
{
"ambientocclusion": false,
"textures": {
"particle": "#torch"
},
"elements": [
{ "from": [ -1, 3.5, 7 ],
"to": [ 1, 13.5, 9 ],
"rotation": { "origin": [ 0, 3.5, 8 ], "axis": "z", "angle": -22.5 },
"shade": false,
"faces": {
"down": { "uv": [ 7, 13, 9, 15 ], "texture": "postap:blocks/torch_i" },
"up": { "uv": [ 7, 6, 9, 8 ], "texture": "postap:blocks/torch_i" }
}
},
{ "from": [ -1, 3.5, 0 ],
"to": [ 1, 19.5, 16 ],
"rotation": { "origin": [ 0, 3.5, 8 ], "axis": "z", "angle": -22.5 },
"shade": false,
"faces": {
"west": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" },
"east": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" }
}
},
{ "from": [ -8, 3.5, 7 ],
"to": [ 8, 19.5, 9 ],
"rotation": { "origin": [ 0, 3.5, 8 ], "axis": "z", "angle": -22.5 },
"shade": false,
"faces": {
"north": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" },
"south": { "uv": [ 0, 0, 16, 16 ], "texture": "postap:blocks/torch_i" }
}
}
]
}